Exercise 10 - Morphology
During this week’s lecture we went through different algorithms for image processing based on mathematical morphology.
Task 1 (Problem 9.6 in Gonzalez and Woods)
Task 2 (Problem 9.17 in Gonzalez and Woods)
Task 3 (Problem 9.27 in Gonzalez and Woods)
Task 4
This exercise aims to provide the necessary knowledge in order to work effectively with morphological operations in python (matlab). For python, we have several options, and you can use whatever appropriate. Some docs can be found here:
and examples how to use them:
In this task, we will use the image circles.png
, which can be found
here, as an example image.
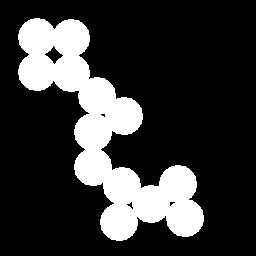
a)
Find the boundary of the circles by using morphological erosion. Try with two different disks as structuring elements, one with radius 1 and one with radius 4. What is the result of using different structure elements?
b)
Find the skeleton of the image (using e.g. skeletonize(img)
from
skimage.morphology
). Note that this function implicitly invokes a sufficient
number of erosions, and can be compared to matlab’s bwmorph(img, 'skel', inf)
.
c)
Generate a half checkerboard image like the one shown in Figure 2, e.g. by using the following code snippet.
import numpy as np
def checkerboard(board_height, board_width, square_size, c1=0, c2=255):
tile2x2 = np.array([[c1, c2], [c2, c1]]).repeat(square_size, axis=0).repeat(square_size, axis=1)
grid = np.tile(tile2x2, (board_height/2, board_width/2))
return grid
chess_board = checkerboard(10, 10, 10, 0, 1)
half_chess_board = np.copy(chess_board)
half_chess_board[:, 50:] = 0
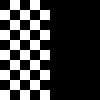
Perform a hit-or-miss transform on the half checkerboard image. Here, you can use the snippet
from scipy import ndimage
s1 = np.array([[0, 0, 0], [1, 1, 0], [0, 1, 0]])
s2 = np.array([[0, 1, 1], [0, 0, 1], [0, 0, 0]])
hom_transformed = ndimage.binary_hit_or_miss(half_chess_board, structure1=s1,
structure2=s2).astype(np.int)
Here, structure1
is a structuring element where non-zero elements defines the
pattern that has to hit the pattern in the input image. Conversly, structure2
is a
structuring element which non-zero elements defines the pattern that has to
miss the pattern in the input image. Use the following hit and miss
structuring elements
Answer this question before you test it:
- Which pattern do you detect by performing a hit and miss with this interval on the half checkerboard image?
Then, aswer the following
- Did it match?
- What happens in the middle of the checkerboard pattern?
- How should the structuring elements be designed in order to detect the top left corner of all the white squares?
Task 5
In inspection of electronic circuit cards there is a need to inspect the number of holes and the diameter of the holes, see Figure 3 (the image is to be found here).
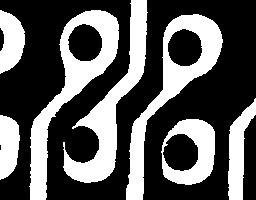
We want to measure the number of holes and their diameter using morphology.
Task 6
Experiment with using either top hat or bottom hat as a preprocessing before
applying global thresholding to the image morf_test.png
, which can be found
here. This image contain letters on a very noisy
background with varying illumination and is displayed in Figure 4.
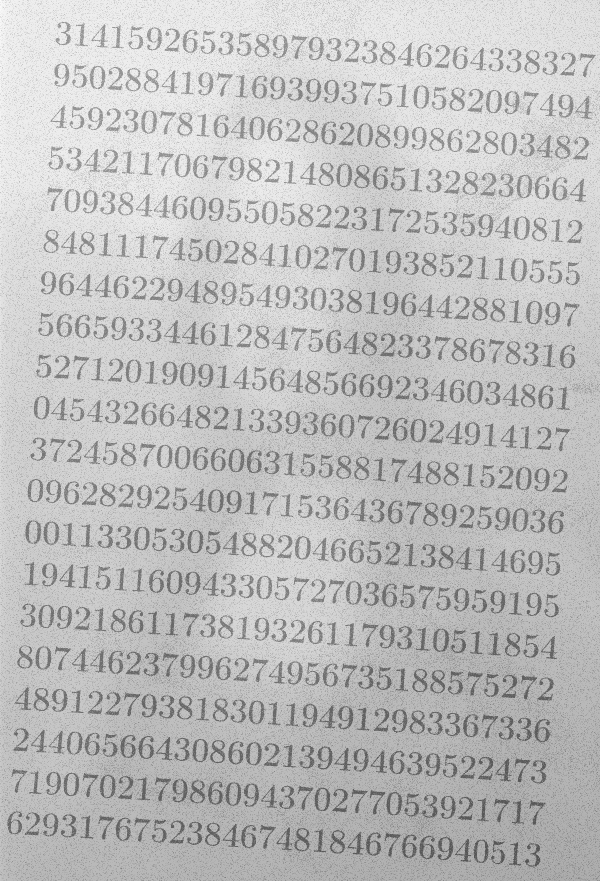
Try to compute a good estimate of the background, and subtract the estimated background from the original. Then try to use global thresholding on the resulting image. Do you also need a noise filter? Can you segment out the numbers fairly well?
Also consider if there are gaps or connected symbols that you can improve by applying simple binary morphological operations.